- Get started
- Flutter Layout Explorer
- Using the Layout Explorer
Note: The inspector works with all Flutter applications.
What is it?
How to install Flutter in Visual Studio Code and run Android EmulatorFlutter installation steps with Visual Studio Code:1, Download Android Studio 20202,Dow. Setting up Flutter and Visual Studio Code In Windows. Detailed video with all the proper steps to setup Flutter and to create a proper working environment fo. From version 2.13.0 of Dart Code, emulators can be launched directly from within Visual Studio Code, but this feature relies on support from the Flutter tools which means it will only show emulators when using a very recent Flutter SDK. Flutter is Google’s UI toolkit for building beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. Flutter - Flutter support and debugger for Visual Studio Code. Flutter Color - A user friendly plugin to help you work with ARGB Color in VS Code, for any project. Flutter Snippets - Supercharge your Flutter workflow with handy Snippets for VS Code Pubspec Assist - Easily add dependencies to your Dart /.
The Flutter widget inspector is a powerful tool for visualizing andexploring Flutter widget trees. The Flutter framework uses widgetsas the core building block for anything from controls(such as text, buttons, and toggles),to layout (such as centering, padding, rows, and columns).The inspector helps you visualize and explore Flutter widgettrees, and can be used for the following:
- understanding existing layouts
- diagnosing layout issues
Get started
To debug a layout issue, run the app in debug mode andopen the inspector by clicking the Flutter Inspectortab on the DevTools toolbar.
Note: You can still access the Flutter inspector directly from Android Studio/IntelliJ, but you might prefer the more spacious view when running it from DevTools in a browser.
Debugging layout issues visually
The following is a guide to the features available in theinspector’s toolbar. When space is limited, the icon isused as the visual version of the label.
Enable this button in order to select a widget on the device to inspect it. For more information, see Inspecting a widget.
Inspecting a widget
You can browse the interactive widget tree to view nearbywidgets and see their field values.
To locate individual UI elements in the widget tree,click the Select Widget Mode button in the toolbar.This puts the app on the device into a “widget select” mode.Click any widget in the app’s UI; this selects the widget on theapp’s screen, and scrolls the widget tree to the corresponding node.Toggle the Select Widget Mode button again to exitwidget select mode.
When debugging layout issues, the key fields to look at are thesize
and constraints
fields. The constraints flow down the tree,and the sizes flow back up. For more information on how this works,see Understanding constraints.
Flutter Layout Explorer
The Flutter Layout Explorer helps you to better understandFlutter layouts.
For an overview of what you can do with this tool, seethe Flutter Explorer video:
You might also find the following step-by-step article useful:
Using the Layout Explorer
From the Flutter Inspector, select a widget. The Layout Explorersupports both flex layouts and fixed size layouts, and hasspecific tooling for both kinds.
Flex layouts
When you select a flex widget (for example, Row
, Column
, Flex
)or a direct child of a flex widget, the flex layout tool willappear in the Layout Explorer.
The Layout Explorer visualizes how Flex
widgets and theirchildren are laid out. The explorer identifies the main axisand cross axis, as well as the current alignment for each(for example, start, end, and spaceBetween).It also shows details like flex factor, flex fit, and layoutconstraints.
Additionally, the explorer shows layout constraint violationsand render overflow errors. Violated layout constraintsare colored red, and overflow errors are presented in thestandard “yellow-tape” pattern, as you might see on a runningdevice. These visualizations aim to improve understanding ofwhy overflow errors occur as well as how to fix them.
Clicking a widget in the layout explorer mirrorsthe selection on the on-device inspector. Select Widget Modeneeds to be enabled for this. To enable it,click on the Select Widget Mode button in the inspector.
For some properties, like flex factor, flex fit, and alignment,you can modify the value via dropdown lists in the explorer.When modifying a widget property, you see the new value reflectednot only in the Layout Explorer, but also on thedevice running your Flutter app. The explorer animateson property changes so that the effect of the change is clear.Widget property changes made from the layout explorer don’tmodify your source code and are reverted on hot reload.
Interactive Properties
Layout Explorer supports modifying mainAxisAlignment
,crossAxisAlignment
, and FlexParentData.flex
.In the future, we may add support for additional propertiessuch as mainAxisSize
, textDirection
, andFlexParentData.fit
.
mainAxisAlignment
Supported values:
MainAxisAlignment.start
MainAxisAlignment.end
MainAxisAlignment.center
MainAxisAlignment.spaceBetween
MainAxisAlignment.spaceAround
MainAxisAlignment.spaceEvenly
crossAxisAlignment
Supported values:
CrossAxisAlignment.start
CrossAxisAlignment.center
CrossAxisAlignment.end
CrossAxisAlignment.stretch
FlexParentData.flex
Layout Explorer supports 7 flex options in the UI(null, 0, 1, 2, 3, 4, 5), but technically the flexfactor of a flex widget’s child can be any int.
/avdmanager.png)
Flexible.fit
Layout Explorer supports the two different types ofFlexFit
: loose
and tight
.
Fixed size layouts
When you select a fixed size widget that is not a childof a flex widget, fixed size layout information will appearin the Layout Explorer. You can see size, constraint, and paddinginformation for both the selected widget and its nearest upstreamRenderObject.
Details Tree
Select the Details Tree tab to display the details tree for theselected widget.
From the details tree, you can gather useful information about awidget’s properties, render object, and children.
Track widget creation
Part of the functionality of the Flutter inspector is based oninstrumenting the application code in order to better understandthe source locations where widgets are created. The sourceinstrumentation allows the Flutter inspector to present thewidget tree in a manner similar to how the UI was definedin your source code. Without it, the tree of nodes in thewidget tree are much deeper, and it can be more difficult tounderstand how the runtime widget hierarchy corresponds toyour application’s UI.
You can disable this feature by passing --no-track-widget-creation
tothe flutter run
command.
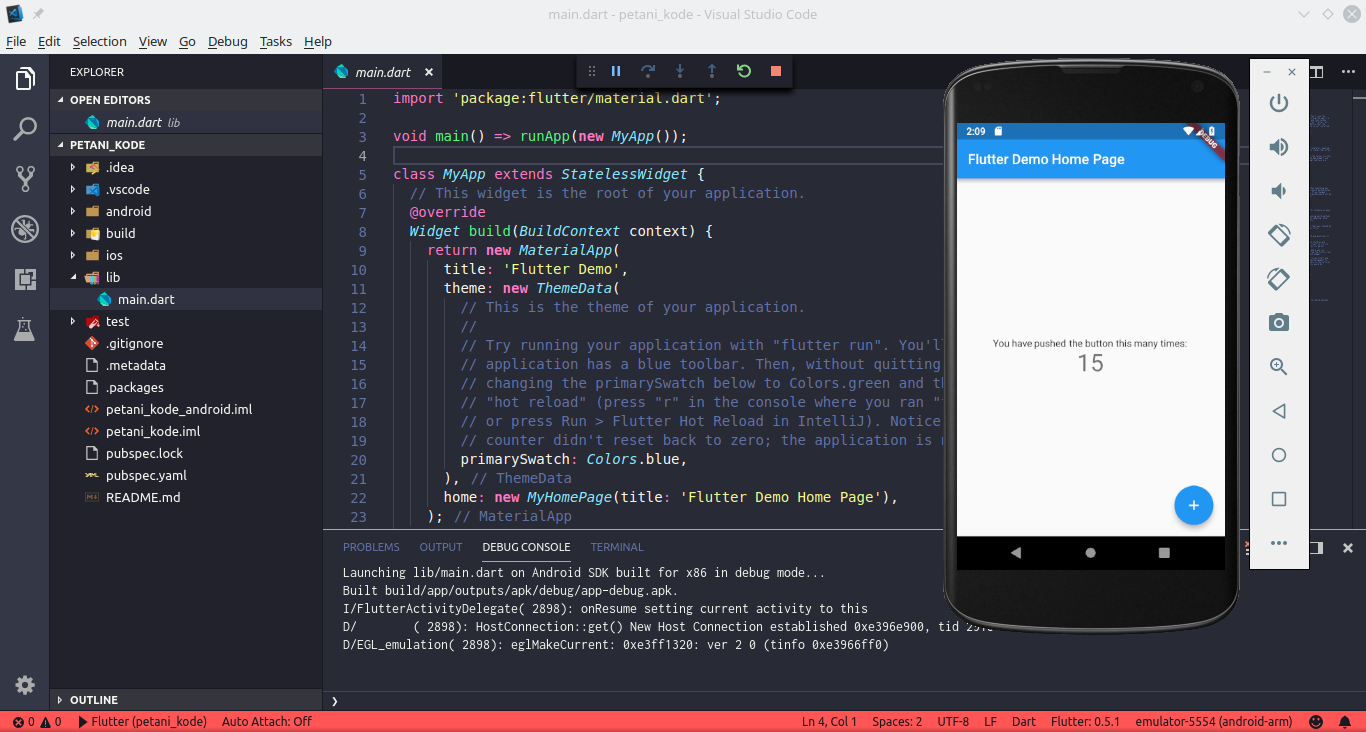
Here are examples of what your widget tree might look likewith and without track widget creation enabled.
Track widget creation enabled (default):
Track widget creation disabled (not recommended):
This feature prevents otherwise-identical const
Widgets frombeing considered equal in debug builds. For more details, seethe discussion on common problems when debugging.
Visual Studio Code Flutter Setup
Other resources
For a demonstration of what’s generally possible with the inspector,see the DartConf 2018 talk demonstrating the IntelliJ versionof the Flutter inspector.
While your code might follow any preferred style—in ourexperience—teams of developers might find it more productive to:
- Have a single, shared style, and
- Enforce this style through automatic formatting.
The alternative is often tiring formatting debates during code reviews,where time might be better spent on code behavior rather than code style.
Automatically formatting code in Android Studio and IntelliJ
Install the Dart
plugin (see Editor setup)to get automatic formatting of code in Android Studio and IntelliJ.To automatically format your code in the current source code window,use Cmd+Alt+L
(on Mac) or Ctrl+Alt+L
(on Windows and Linux).Android Studio and IntelliJ also provides a check box named Format code on save
onthe Flutter page in Preferences (on Mac) or Settings (on Windows and Linux)which will format the current file automatically when you save it.
Automatically formatting code in VS Code
Install the Flutter
extension (see Editor setup)to get automatic formatting of code in VS Code.
To automatically format the code in the current source code window,right-click in the code window and select Format Document
.You can add a keyboard shortcut to this VS Code Preferences.
To automatically format code whenever you save a file, set theeditor.formatOnSave
setting to true
.
Automatically formatting code with the ‘flutter’ command
You can also automatically format code in the command line interface(CLI) using the flutter format
command:
Using trailing commas
Flutter code often involves building fairly deep tree-shaped data structures,for example in a build
method. To get good automatic formatting,we recommend you adopt the optional trailing commas.The guideline for adding a trailing comma is simple: Alwaysadd a trailing comma at the end of a parameter list infunctions, methods, and constructors where you care aboutkeeping the formatting you crafted.This helps the automatic formatter to insert an appropriateamount of line breaks for Flutter-style code.
Flutter In Visual Studio Code
Here is an example of automatically formatted code with trailing commas:
And the same code automatically formatted code without trailing commas:
